Core Java Interview Questions and Answers
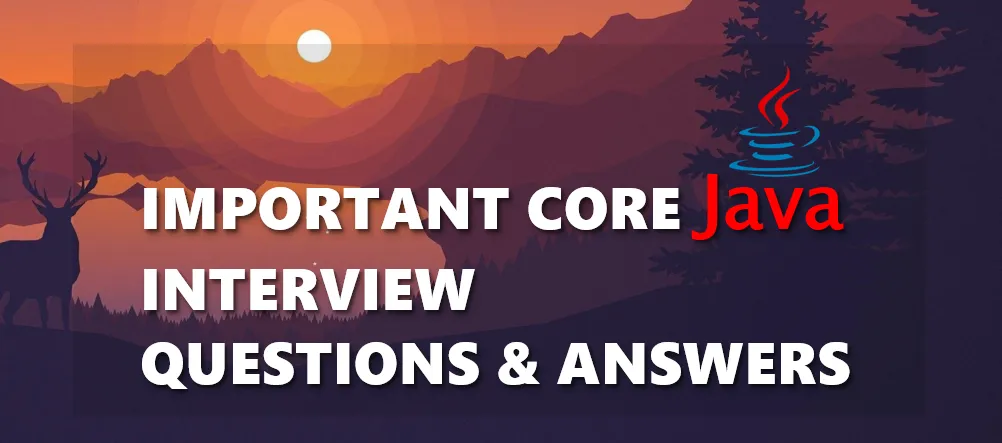
This is a Collection of very important core java interview questions with answers.
These questions will help students and job seekers to gain confidence to appear and get success in java interviews.
Lot of importance is given to a candidate’s ability to answer core java questions in a java job interview.
For private Online Core Java Training with java interview preparation you may contact me.
Top 15 Frequently Asked Core Java Interview Questions
- What is a class?
- What is an object?
- Explain garbage collection in java.
- What is function overloading?
- What is a Constructor and its use?
- What is static keyword in java?
- Explain run time polymorphism.
- What are the differences between an abstract class and an interface?
- Explain the use of throw and throws keyword.
- What is the difference between checked and unchecked exceptions?
- Explain about different access specifiers.
- What is Synchronization?
- How to Create Multiple Threads?
- What is difference between Path and CLASSPATH?
- How does the Object-Oriented approach improve software development?
1. What is a Class?
A class is a user defined datatype. It is a frame of all the objects that can be created using it. A class can contain data properties and functional properties.
Example
1 2 3 4 5 6 7 8 | class Soldier { int x,y;// instance variables declaration void fire()//function definition { System.out.println("Firing from "+x+" "+y); } } |
2. What is an object?
An object is an instance of a class. Each object will contain its own copies of the instance variables and the functions defined in a class. To create an object we use the new keyword.
- Soldier s1=new Soldier();
new Soldier() will create an object and make s1 is a reference variable of Soldier type that refers to the Soldier object created.
3. What is difference between instance variable and reference variable?
The data properties in a class are declared with variables. These variables are called instance variables. Example in the Soldier class we have declared two data properties the x coordinate and the y coordinate representing the location of a soldier. These data properties are called instance variables.
Each object of the Soldier class will have its own copies of the instance variable. e.g the first soldier object will have its own x and y and the second soldier object will have its own copy of x and y.
Reference variable is the variable that can refer to objects. Eg Soldier s1 is a Soldier type reference variable that can refer to Soldier type of objects.
4. Explain garbage collection in java.
When an object in the memory doesn’t have any reference variable pointing to it, java considers it a useless object, unnecessarily occupying memory. Java periodically searches for such type of hanging objects and removes them from memory. This procedure is called automatic garbage collection. We can tell the jvm to start the garbage collection by calling the function System.gc()
5. What is function overloading?
Function overloading is one type of polymorphism in which the function name is same but the parameter list is different. The number or nature of the parameters may be different.
6. What is a Constructor and its use?
A Constructor is just like a function with the same name as the class name and no return type, not even void. Constructors are generally used to initialize instance variables during object creation.
7. What is this keyword in java?
The keyword “this” refers to the current class object.
8. What is super keyword in java?
The “super” keyword in java refers to the parent class object.
9. What is static keyword in java?
The keyword static can be put in front of a variable , function or a block in java. To access a static variable or a static function we don’t need to create an object. We cann access a static variable or a function directly through the class name e.g classname.function() or classname.variable.
A static variable is shared among all objects of the class.
10. Can we make the constructor of a class static?
We know that the static context (methods, blocks, variables) belongs to the class and not the object. Constructors can only be invoked when an object is created. Therefore, it makes no sense to make constructors static. The compiler will alert you if this is attempted.
11. Can static methods be overridden?
No we cannot override static methods. But we can overload static methods.
12. What is the significance of the main function being static?
The function main is the starting point of program execution. There is no need for the jvm to create an object of the class to access the main function. So the main function is made static.
13. What happens if the static keyword is not included in the main method signature in Java?
If we don’t include the ‘static’ keyword in the main method definition, the compilation of the program will go through without any issues but when we’ll try to execute it, a “NoSuchMethodError” error will be thrown.
14. What is the use of inheritance in programming?
The main benefit of inheritance is code reusability. The properties of the parent class are available in the child classes. The child class inherit from the parent class using the extends keyword.
15. Does java support multiple inheritance?
Java doesn’t support multiple inheritance through classes but supports through interfaces. Class A extends B,C is not possible but class A implements X,Y is possible provided X and Y are interfaces.
16. What is difference between Path and CLASSPATH?
Path is an environment variable that guides the operating system from where it will search for executable files.
CLASSPATH is an environment variable that helps to educate the JVM from where it will start searching for .class files. generally we store the root of the package heirarchy in the CLASSPATH environment variable.
We also store the location of external jar files in the environment variable, so that the jvm can locate the class files within it.
17. What is function overriding?
Same function name and same parameter list, and can happen during inheritance only.
18. Explain run time polymorphism.
Java resolves dynamically at run time, which function to call, based on which sub class is referred by the super class reference variable just before the function is called.
Figure f=new Rectangle(); f.area();
In the above code the area of the rectangle class get called because just before the function is called, the reference variable is pointing to a Rectangle object.
19. What is an abstract class?
Any class can be made abstract by just putting the abstract keyword in front of the class. But if a class contains one or more abstract functions, we are bound to make the class abstract.
We cannot create an object of an abstract class.
Abstract classes are generally used to create the frame of the project, or the top level class.
Subclass of an abstract class has to provide implementation of all the abstract functions present in the super class, else the subclass will also become abstract.
We can also implement run time polymorphism through abstract classes.
20. What is Object class?
Object
class in Java is the root of the class hierarchy. Every class in Java, either directly or indirectly, inherits from Object
. It provides a set of important methods that all Java objects can use, such as equals()
, hashCode()
, toString()
, clone()
, getClass()
, finalize()
, wait()
, notify()
, and notifyAll()
. These methods help with fundamental operations like object comparison, memory management, and thread synchronization.
This class is implicitly extended by every other class, ensuring that all Java objects share a common set of behaviors
Object class is an inbuilt class present in the java.lang package. It is the super class of all classes in java.
Whenever we create a class, by default it is the subclass of the object class. We can override non final functions of the Object class in any class eg toString(), equals() etc.
21. What is the use of toString function?
The toString function gets automatically inherited in all java classes from the Object class. We can override the toString function. Whenever we print an object of a class, by default the toString function of the class gets called. So if we want to give a customizable output when printing an object, we can override the toString function.
22. Explain about different access specifiers.
The different access specifiers are public, private, protected and the default.
private properties are accessible from only within the same class.
public properties are accessible from anywhere.
no modifier properties are accessible only from within the same package.
protected properties are accessible from the same package, but out side the package it is accessible provided it is done from a sub class.
23. What are the differences between an abstract class and an interface?
An abstract class can contain both function definitions and function declarations, but an interface can contain only function declarations.
An abstract class is extended by using the extends keyword while an interface is implemented using the implements keyword.
Multiple inheritance is not supported through classes or abstract classes but is supported through interfaces.
24. What is final keyword in java?
The final keyword in java can be used Infront of a variable, function or a class.
If a final keyword is placed Infront of a variable, it acts like a constant. It cannot be modified.
Eg final int YES=100;
By convention final variables are in upper case. They are declared and initialized in the same line.
Function defined as final cannot be overridden in the subclasses.
Classes defined as final cannot be subclassed or inherited.
25. What are wrapper classes, and their use?
Java provides special classes corresponding to each of the primitive data types. These are called wrapper classes. They are e.g. Integer, Character, Double etc.
Most of the collection classes store objects and not primitive data types. Wrapper classes also provide many utility methods. And since we create instances of these classes we can store them in any of the collection classes and pass them as a collection. Also we can pass them as method arguments where a method expects an object.
26. What is an exception in java?
Exceptions are run time errors. e.g. int i=1/0; This code doesn’t show any error during compile time, but when we run the code we get an ArithmeticException.
27. What is exception handling?
When we run a program that has error, java creates an appropriate exception type of object and throws it. Then the default exception handler of java handles the exception. But the default exception handler stops the program execution abruptly and gives a non understandable output.
So we are not satisfied by the default exception handler’s exception handling. So we want to handle the exception on our own so that the program will not stop abruptly and also give a non understandable output. We can do exception handling on our own using try and catch block.
28. What is the use of printStackTrace() method?
It helps to trace the exception step by step. It helps to find how the exception occurred step by step.
29. Explain custom exception class.
We need a custom exception class to represent a custom problem. There are many inbuilt exception classes in java like ArithmeticException that represents arithmetic problems, ArrayIndexOutOfBounds exception to represent array related problems. But we need custom exception classes to represent custom problems, or our own problems. e.g. ProductNotFoundException is a custom exception class representing a problem which occurs if a product is not found during the search. To create a custom exception class we have to extend the inbuilt Exception class.
30. Explain the use of throw keyword.
The throw key word is used to manually throw exception objects. If there is a division by zero or array problem java creates an appropriate exception object and throws it. But if there is a custom problem, java will not throw the exception but we have to manually throw the exception objects on our own using the throw keyword.
e.g
1 | if(productNotFound) throw new NoProductFoundException(); |
Here NoProductFoundException is a custom exception class. If product is not found then we are manually creating an object of NoProductFoundException class and throwing it using throw keyword.
31. What is the use of throws keyword?
The throws keyword is used to delegate the exception handling mechanism to the caller of the function. When we define a function and we don’t want to put try and catch block than we can use throws keyword. The caller of the function has to put the try and catch blocks, or handel the exception.
eg.
1 | static void fun() throws ProductNotFoundException |
32. What is the difference between checked and unchecked exceptions?
Checked exceptions are those exceptions that cannot be handled by the default exception handler of java. So we have to manually handle the exception using try catch block. All the custom exceptions are checked exceptions.
Unchecked exceptions are those exceptions that can be handled by the default exception handler of java. eg ArithmeticException, NullpointerException etc.
33. What is Thread in Java?
A thread is a light weight process. Using thread multiple activities can be done within a single process. Thread based multitasking is called multithreading. Thread is an inbuilt class in java in the java.lang package.
34. What is Multithreading and its advantage?
Thread based multitasking is called multithreading. Main advantages of using multithreading are
- Multiple things can be done simultaneously in a process.
- Maximum utilization of the cpu. Cpu is very fast as compared to Input Output. When one thread is idle, another thread gets chance.
35. How to Create Multiple Threads?
There are two ways to create multiple threads. By implementing the Runnable interface or extending the Thread class.
Using implementing Runnable technique the steps are as follows:
1: Create a child class that implements the runnable interface.
2: Provide the working of the thread inside the run method
3: Create another class containing the main function.
4: Inside the main, create an object of the child class and pass it into the threads constructor.
5: Then the start() function on the thread object is called. This will invoke the run method defined
in the child class.
Check Detail Example >> How to Create Threads in Java
36. What is Synchronization?
Synchronization is a way to ensure that only one thread can access a resource at a particular time. Synchronization can be done using two way. Method level synchronization, and object level synchronization. Method level synchronization can be done by putting synchronized keyword in front of a function. Object level synchronization can be done through the help of synchronized block.
37. Which functions are used for inter thread communication?
Inter thread communication can be done in java using the functions wait(), notify() and notifyAll().
wait() function will make a thread to go to sleep until it is woken up using notify() function.
notify() function will wake up the the recent thread on whom wait() had been called.
notifyAll() function will wake up all the previous threads on whom wait() had been called.
38. Explain System.out.println()
System is an inbuilt class in java. “out” is a PrintStream type of stream object connected with the console, and println() is a function present in the PrintStream class. So when we want to display something in the console we use System.out.println().
39. What is Java Virtual Machine or JVM?
The JVM or Java Virtual Machine is a virtual machine that runs java files in a portable way. It is a specification that provides runtime environment in which java byte code can be executed. Jvm calls the main method present in a java code. It is a part of the JRE(Java Runtime Environment). JVM helps to make java code platform independent.
40. What is Jit Compiler?
Jit Compiler or “Just in Time Compiler” is an essential part of the JRE, that improves the performance of Java applications. It compiles bytecode to native machine code at run time. The JIT compiler is able to perform certain simple optimizations while compiling a series of bytecode to native machine language.
41. Is java a pure object oriented language?
No java is not a pure object oriented language because it also supports primitive data types like byte, boolean, char, short, int, float, long, and double.
42. What is the base class of all exception classes?
There is an inbuilt class in java i.e java.lang.Throwable, it is the super class of all other exception classes in java.
42. Can we call a non static function from within a static function?
No, non static need to be called through object references. We can call static functions from within a static method. To access non static functions in java we need to create objects and access through the object. But to access a static functions we don’t need to create objects, we can directly access through the class name.
43. How does the Object-Oriented approach improve software development?
The key benefits are:
Reusability of previous work: using inheritance and object composition.
Mapping to the problem domain: Objects map to real world and represent products, bank account, vehicles, customers, etc: using encapsulation.
Modular Architecture: Objects, systems, frameworks etc are the building blocks of larger systems.
The above key benefits lead to increase in software quality and decrease in software development time. If 90% of the new application consists of proven existing components then only the remaining 10% of the code has to be developed and tested.
43. Explain the Singleton Design Pattern with an example.
The Singleton pattern ensures a class has only one instance and provides global access to it.
1 2 3 4 5 6 7 8 | public class Singleton { private static Singleton instance; private Singleton() {} public static Singleton getInstance() { if (instance == null) instance = new Singleton(); return instance; } } |
44. What is the difference between String
, StringBuilder
, and StringBuffer
?
In Java, String is immutable, meaning its value cannot be changed once created. If modified, it creates a new object and changes the reference. Whereas StringBuilder is mutable and allows modifications without creating new objects, making it faster but not thread-safe. On the other hand, StringBuffer is also mutable and provides thread safety, making it suitable for multi-threaded environments but slightly slower than StringBuilder due to synchronization overhead.
45. What is the difference between shallow copy and deep copy?
In shallow copy, the references of objects are copied instead of creating new independent copies. As a result, any changes made to the original object will be reflected in the copied object since both share the same memory location, whereas in deep copy, completely independent copies of objects are created, including nested objects. Consequently, changes in the original object do not affect the copied object, as they exist in separate memory locations.
46. How does the Comparator
interface differ from Comparable
?
- Comparable: Used for natural ordering; implements
compareTo()
. - Comparator: Used for custom ordering; implements
compare()
.
1 2 3 4 | class Student implements Comparable<Student> { int id; public int compareTo(Student s) { return this.id - s.id; } } |
47. What is a marker interface?
A marker interface is an empty interface (no methods) used to signal to the JVM or compiler that a class possesses a certain property. Examples: Serializable
, Cloneable
.
43. What is the purpose of the volatile
keyword in Java?
The volatile
keyword ensures visibility of changes to variables across threads. It prevents threads from caching variables.
1 | private volatile boolean running = true; |
44. What is a record
in Java, and how does it differ from a regular class?
A record in Java is a special type of class introduced in Java 14 to create immutable data objects with less boilerplate code. Unlike regular classes, records automatically generate a constructor, getters, equals()
, hashCode()
, and toString()
methods based on their fields. All fields are final, so it can’t be changed once set.
The key difference from a regular class is that records are focused purely on holding data and can’t extend other classes. They’re best suited for simple data containers like Data Transfer Objects (DTOs) or cases where immutability is essential. Regular classes are better when you need mutable fields, complex inheritance, or you may need more logic and customization.
Using a Record:
1 | public record Person(String name, int age) {} |
Records are perfect for simple, immutable data objects and help keep your code clean and concise.
Using a Regular Class:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | public class Person { private final String name; private final int age; public Person(String name, int age) { this.name = name; this.age = age; } public String getName() { return name; } public int getAge() { return age; } @Override public boolean equals(Object o) { /* Manually implement equals */ } @Override public int hashCode() { /* Manually implement hashCode */ } @Override public String toString() { return "Person{name='" + name + "', age=" + age + "}"; } } |
45. What is a Functional Interface in Java, and how is it different from a regular interface?
A Functional Interface in Java is an interface that has exactly one abstract method. It can have default and static methods, but only one abstract method. It is mainly used for Lambda Expressions and Method References.
1 2 3 4 | @FunctionalInterface interface Log { void logMessage(String message); // Single abstract method } |
46. Will adding @FunctionalInterface
cause an error if there are multiple abstract methods? And is this annotation necessary to make an interface functional?
Yes, if an interface is marked with @FunctionalInterface and has more than one abstract method, the compiler will throw an error. However, the annotation itself is not required, an interface with only one abstract method automatically behaves as a functional interface, even without it.
So @FunctionalInterface only prevents accidental addition of extra abstract methods. It also makes the intention clear that this interface is meant for functional programming.
47. What are the different ways to implement a Functional Interface, and which one is preferred?
There are a few ways to implement a functional interface in Java. The traditional way is by using an anonymous inner class, where we create an instance and directly implement the abstract method. We can also directly implement the interface, but that approach tends to be more verbose.
A cleaner and more modern approach is through lambda expressions. Since functional interfaces have a single abstract method, lambdas make the code much more concise and readable.
Then we have method references, which act as a shortcut when the lambda simply calls an existing method. It’s even more compact and improves readability.
Out of these, lambda expressions and method references are preferred in most cases because they make the code cleaner, more maintainable, and less cluttered. Method references are ideal when there’s already an existing method that matches the functional interface’s method signature.
48. What are some common built-in functional interfaces in Java, and can you give examples of their abstract methods?
Some common built-in functional interfaces in Java are:
- Runnable : This functional interface represents a task that takes no input and returns no result. Its abstract method is
run()
. We often use it for multithreading. - Predicate<T> / BiPredicate<T, U> : It is used for conditional checks. Predicate’s abstract method is
test(T t)
, while BiPredicate’s istest(T t, U u)
for two inputs. They are commonly used for filtering data or comparing values. - Function<T, R> / BiFunction<T, U, R> : This one is used for transforming data. Function has
apply(T t)
for single input, while BiFunction extends it for two inputs withapply(T t, U u)
. A common use case is data transformations. - Consumer<T> : Performs operations without returning a result, using
accept(T t)
. For example, printing elements from a list. - Supplier<T> : Generates a value without input using
get()
. A common example is fetching random values.
49. Can you explain the difference between Callable
and Runnable
in Java and when you would use each?
Callable and Runnable are both used for multithreading in Java but serve different purposes. Runnable is suitable for tasks that don’t need to return a result or throw checked exceptions. It’s good for simple operations like logging.
On the other hand, Callable is more flexible. It can return a result and throw checked exceptions, making it a better fit for tasks that require a computed result or error handling, such as fetching data or performing calculations. We use it often with ExecutorService to submit tasks and retrieve results using Future objects.
If I don’t need a result or exception handling, Runnable is sufficient. But when a result or error handling is necessary, Callable is the better choice for us.
50. What are the different mechanisms by which threads can acquire locks in Java to ensure safe access to shared resources?
Java offers various locking mechanisms to ensure thread-safe access to shared resources.
- Intrinsic Locks (synchronized) automatically acquire and release locks when threads enter and exit synchronized blocks or methods, providing a straightforward approach to thread synchronization.
- However, for more advanced control, Explicit Locks (ReentrantLock) offer methods like
lock()
andunlock()
, along with additional features such as try-locking and interruption handling. - When performance optimization is essential, Read-Write Locks (ReadWriteLock) become valuable. They allow multiple readers or a single writer, making them ideal for scenarios where reads are more frequent than writes.
- We also have Stamped Locks (StampedLock), which take concurrency a step further by introducing optimistic, read, and write locks. These locks enable non-blocking reads, making them well-suited for conditions where read operations are frequent, while write operations are minimal.
Please check again we are updating this with more Core Java interview questions
51. What is the difference between deadlock and livelock in multithreading?
In a deadlock, two or more threads are stuck waiting for each other to release resources,. This often happens due to holding locks, and none of them can proceed. This generally occur when threads acquire resources in different orders or fail to release them properly. We can be resolved by carefully ordering resource requests, using timeout mechanisms, or by applying deadlock detection techniques.
On the other hand, a livelock occurs when threads keep responding to each other’s actions but fail to make progress. Although the threads remain active, they are essentially stuck in a continuous loop of state changes. Livelocks can be prevented by introducing randomness or conditions that eventually break the loop.
So, in short, deadlock is about being stuck without action, while livelock is about constant action without progress.
52. What is a race condition in multithreading, and how can it be prevented?
A race condition occurs in multithreading when two or more threads try to access and modify shared resources at the same time, this lead to unpredictable or incorrect behavior.
To prevent race conditions, we can use synchronization mechanisms such as locks, synchronized blocks/methods, or atomic variables. These ensure that only one thread can access the shared resource at a time, preserving data integrity.
53. What is a memory leak, and how does it affect garbage collection?
A memory leak in Java occurs when unused objects remain referenced, preventing garbage collection. This leads to increased memory usage, degraded performance, and potential system failure. Common causes include not closing resources, holding unnecessary references, and excessive object creation. So, using proper resource and reference management help us prevent memory leaks.
54. What is Reflection Pooling?
Reflection pooling in Java is an optimization technique where reflective objects (such as Method, Field, Constructor, etc.) are cached and reused instead of being created repeatedly. Since reflection is a slow and expensive operation due to runtime type inspection and security checks, maintaining a pool of frequently accessed reflective objects helps reduce overhead and improve performance.
55. What is ExecutorService?
ExecutorService is a high level Java concurrency API provided by the java.util.concurrent package that simplifies thread management. It provides a pool of worker threads to execute tasks asynchronously, improving performance by reusing threads instead of creating new ones repeatedly.
56. What is the difference between optimistic locking and pessimistic locking in Java? Provide a real-world example. **
Optimistic locking assumes no conflicts and allows multiple transactions to proceed without locking. It checks for conflicts only at the time of update, usually using a version number or timestamp. If a conflict is detected, the transaction retries. This is useful in high-read, low-write scenarios like online ticket booking.
Pessimistic locking, on the other hand, locks the resource at the start of a transaction, preventing others from accessing it until the lock is released. This ensures data consistency but reduces concurrency. It’s used in banking systems to prevent double withdrawals from the same account.
58. What is the Vector class in Java’s Collection framework, when was it introduced, and is it still commonly used today? If not, what are the preferred alternatives?
The Vector
class is a legacy collection in Java that implements a growable array, similar to ArrayList
, but with synchronized methods for thread safety. It was introduced in Java 1.0, before the Collection framework.
Today, Vector
isn’t commonly used because its synchronization is often unnecessary, making it slower. Instead, ArrayList
is preferred for non-thread-safe operations, and CopyOnWriteArrayList
or Collections.synchronizedList()
for thread-safe scenarios
59. What is the difference between HashMap and LinkedHashMap?
The main difference is that HashMap
doesn’t maintain any order of elements, while LinkedHashMap
preserves insertion order.
Both allow null
keys and values, but LinkedHashMap
uses a doubly linked list alongside the hash table, making it slightly slower due to extra memory overhead. If ordering matters, go for LinkedHashMap
; otherwise, HashMap
is more efficient.
60. Why do we implement the Serializable interface in some classes?
We implement Serializable to convert objects into a byte stream for storage or transmission. It’s a marker interface, meaning no methods – just a signal to the JVM that the class can be serialized. This helps in saving objects to files, sending them over networks, or caching data. But we should be cautious with sensitive data and versioning.
61. What are the advantages of using generics?
Generics in Java come with several advantages. They ensure type safety by catching errors at compile time, like preventing an Integer
from being added to a List<String>
.
They also eliminate the need for explicit type casting, making code cleaner and reducing ClassCastException
risks.
Lastly, generics improve reusability, allowing us to write a single method or class that works with different types.
So, Overall, they make code more readable, maintainable, and reliable
62. Is the statement ‘Overriding is an example of runtime polymorphism’ true?
It’s partially true. Overriding enables runtime polymorphism, but it isn’t runtime polymorphism itself. True runtime polymorphism happens when a parent reference calls an overridden method of a child object. The method is chosen at runtime based on the actual object’s type, not the reference type. This is called dynamic method dispatch.
Leave a Reply