Recursion in Java
In this tutorial we will see how to do recursion in java, and also see examples of recursion using java.
A recursive method in Java is a method that calls itself, and this process is known as recursion. Recursion in java provides a way to break complicated problems down into simple problems which are easier to solve.
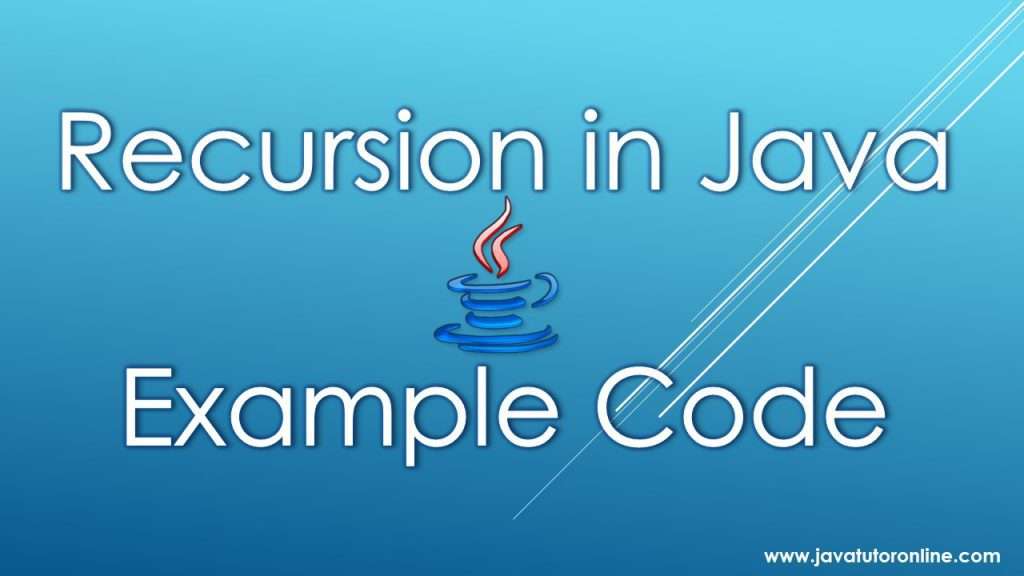
Recursion although a tricky concept is very important topic for java programmers. Online Java Tutor can guide through personalized java training and help students understand basics and advance java coding.
Ten Examples of Recursion in Java
- Factorial of a Number Using Recursion
- Fibonacci Series using Recursion
- Using Java Recursion to Reverse a given String
- Check if a given string is a Palindrome using Recursion
- Find minimum number in an int array using Java Recursion
- Find the number of odd numbers in an int array using Recursion
- Find the largest even int in an int array using Recursion
- Find the sum of numbers larger then a number in an int array using Recursion
- Find the Greatest Common Divisor (GCD) using Recursion
- Implement Binary Search using Recursion
1. Factorial of a Number Using Recursion
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | public class Factorial { public static int factorial(int n) { if (n != 0) // ending condition return n * factorial(n - 1); // recursive call else return 1; } public static void main(String[] args) { int num=7; int fact=factorial(num); System.out.println("Factorial of the number "+num+" is "+fact); } } |
Output -> Factorial of the number 7 is 5040
2. Fibonacci Series using Recursion
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | public class Fibanoccai { public static int fibonacci(int x) { if (x == 0) { return 0; } if (x == 1 || x == 2) { return 1; } return fibonacci(x - 2) + fibonacci(x - 1); } public static void main(String[] args) { int maxNumber = 10; System.out.print("Fibonacci Series of " + maxNumber + " numbers: "); for (int i = 0; i < maxNumber; i++) { System.out.print(fibonacci(i) + " "); } } } |
Output -> Fibonacci Series of 10 numbers: 0 1 1 2 3 5 8 13 21 34
3. Using Java Recursion to Reverse a given String
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | class StringReverse { //recursive method to reverse a given string void reverseString(String str) { //base condition if ((str==null)||(str.length() <= 1)) System.out.println(str); else { System.out.print(str.charAt(str.length()-1)); reverseString(str.substring(0,str.length()-1)); } } } public class Main{ public static void main(String[] args) { String input = "JavaTutorOnline"; System.out.println("The given string: " + input); StringReverse obj = new StringReverse(); System.out.print("The reversed string: "); obj.reverseString(input); } } |
Output
The given string: JavaTutorOnline
The reversed string: enilnOrotuTavaJ
4. Check if a given string is a Palindrome using Recursion
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | public class Palindrome { public static boolean checkPalindrome(String s) { if (s.length() == 0 || s.length() == 1) { return true; } if (s.charAt(0) == s.charAt(s.length() - 1)) { return checkPalindrome(s.substring(1, s.length() - 1)); } return false; } public static void main(String args[]) { String s1="MADAM"; boolean b1=checkPalindrome(s1); if(b1==true) System.out.println("The String \""+s1+"\" is a Palindrome"); else System.out.println("The String \""+s1+"\" is not a Palindrome"); } } |
Output -> The String “MADAM” is a Palindrome
5. Find minimum number in an int array using Java Recursion
1 2 3 4 5 6 7 8 | // function to find minimum number public static int findMin(int[] numbers, int startIndex, int endIndex) { // if size = 0 means whole array // has been traversed if (startIndex == endIndex) return numbers[startIndex]; // stopping condition return Math.min(numbers[endIndex], findMin(numbers, startIndex, endIndex - 1)); } |
6. Find the number of odd numbers in an int array using Recursion
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | // Function to find number of odd integers public static int countOddNumbers(int[] elements, int startIndex, int endIndex) { int count = 0; if (startIndex == endIndex)// stopping condition { if (elements[startIndex] % 2 == 0) { count = count + 0; } else count = count + 1; return count; } else { if (elements[endIndex] % 2 == 0) { count = count + 0; } else count = count + 1; int i = countOddNumbers(elements, startIndex, endIndex - 1) + count; return i; } } |
7. Find the largest even int in an int array using Recursion
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | // Function to find the largest even int public static int computeLargestEven(int[] elements, int startIndex, int endIndex) { int largest = 0; if (startIndex == endIndex) { if (elements[startIndex] % 2 == 0) { largest = elements[startIndex]; } return largest; } else { if (elements[endIndex] % 2 == 0) return Math.max(elements[endIndex], computeLargestEven(elements, startIndex, endIndex - 1)); else return Math.max(0, computeLargestEven(elements, startIndex, endIndex - 1)); } } |
8. Find the sum of numbers larger then a number in an int array using Recursion
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | // Function to find the sum of numbers larger than the first public static int sumOfNumbersLargerThanFirst(int[] elements, int startIndex, int endIndex, int firstNumber) { int count = 0; if (startIndex == endIndex) { if (elements[startIndex] > firstNumber) { count = count + elements[startIndex]; } return count; } else { if (elements[endIndex] > firstNumber) { count = count + elements[endIndex]; } count = count + sumOfNumbersLargerThanFirst(elements, startIndex, endIndex - 1, firstNumber); return count; } } |
9. Find the Greatest Common Divisor (GCD) using Recursion
1 2 3 4 5 6 7 8 9 10 11 12 13 | [crayon-68090679dcdf7138970511 inline="1" lang="java" decode="true" ]public class GCDExample{ public static int gcd(int a, int b) { if (b == 0) { return a; // Base case } return gcd(b, a % b); // Recursive case } public static void main(String[] args) { int a = 48, b = 18; // Example input System.out.println("GCD of " + a + " and " + b + " is: " + gcd(a, b)); } } |
10. Implement Binary Search using Recursion
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | [crayon-68090679dcdfc638829940 inline="1" lang="java" decode="true" ]public class BinarySearchExample { public static int binarySearch(int[] arr, int left, int right, int target) { if (right >= left) { int mid = left + (right - left) / 2; if (arr[mid] == target) { return mid; // Base case: target found } if (arr[mid] > target) { return binarySearch(arr, left, mid - 1, target); // Search in left half } return binarySearch(arr, mid + 1, right, target); // Search in right half } return -1; // Target not found } public static void main(String[] args) { int[] arr = {1, 2, 3, 4, 5}; // Example input int target = 3; int result = binarySearch(arr, 0, arr.length - 1, target); System.out.println("Element found at index: " + result); } } |
11. Decimal to Binary Conversion using Java Recursion
1 2 3 4 5 6 7 8 9 10 11 12 13 | public class DecimalToBinary { public static void toBinary(int n) { if (n == 0) return; // Base case toBinary(n / 2); // Recursive call System.out.print(n % 2); // Print binary digits } public static void main(String[] args) { int number = 10; System.out.print("Binary of " + number + " is: "); toBinary(number); } } |
12. Generate All Subsets of a String using Recursion
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | public class StringSubsets { public static void generateSubsets(String str, String current, int index) { if (index == str.length()) { // Base case System.out.println(current); return; } generateSubsets(str, current, index + 1); // Exclude current character generateSubsets(str, current + str.charAt(index), index + 1); // Include current character } public static void main(String[] args) { String str = "abc"; generateSubsets(str, "", 0); } } |
Main function to call the recursive methods
1 |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 | import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; public class Recursive { public static void main(String[] args) { int arr[] = new int[100]; InputStreamReader isr = new InputStreamReader(System.in); // System.in is a standard input stream object connected with the keyboard BufferedReader br = new BufferedReader(isr); String sr = null; int i = 0;// will keep track of how many int has been entered through the keyboard do { try { sr = br.readLine(); arr[i] = Integer.parseInt(sr); i++; } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } while (!sr.equals("0")); // the array has been populated with values and i is storing number of inputs // calling function to find minimum number int min = findMin(arr, 0, i - 1); System.out.println("The minimum number is " + min); // calling function to find number of odd integers int o = countOddNumbers(arr, 0, i - 1); System.out.println("The count of odd integers in the sequence is " + o); // calling function to find the largest even int int lE = computeLargestEven(arr, 0, i - 1); System.out.println("The largest even integer in the sequence is " + lE); // calling function to find the sum of numbers larger than the first int sum = sumOfNumbersLargerThanFirst(arr, 0, i - 1, arr[0]); System.out.println("The sum of numbers larger than the first is " + sum); } } |
Leave a Reply