Online Core Java Training
This private Core Java Training is focused in developing the students fundamental concepts in Core Java. Training is according to the student’s speed of understanding. Learning Core Java properly and making the basics strong is very important for being good in all java and advance java related technologies. Core Java is used to refer to Java SE, the standard edition.
Core Java Online Training with Live Project helps students to get a hands on working experience. Trainer is a 15 yrs experienced java professional. You may check our Students Testimonial for Online Java Training.
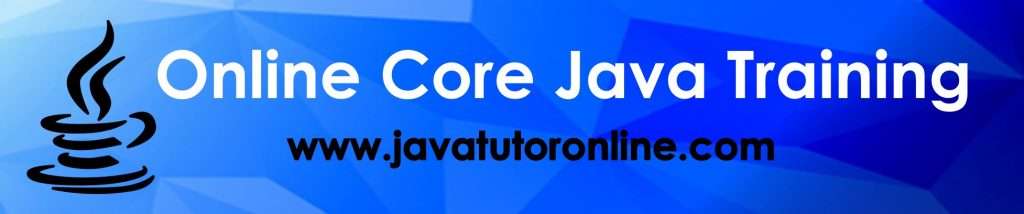
Core Java Training Topics
- Class & Object
- Functions
- Inheritance
- Packages and Interfaces
- Exception Handling
- Multithreading
- Input/Output
- Collection Framework
Sample Core Java Video
Detail List of Topics for Core Java
Below are list of topics that will be covered for core java. Download Eclipse Ide for Java Developers and start learning core java by practically writing code while learning each topic.
Classes and Objects
- Data Types
- Introducing Classes
- Class Fundamentals
- Class Concept through a Real World Scenario
- Instance Variable and Functions
- Creating Objects using new
- Assigning Object Reference Variables
- Garbage Collection
- The finalize( ) Method
A Closer Look at Functions and Classes
- Function Overloading
- Constructors
- Constructors Overloading
- The this Keyword
- Using Objects as Parameters
- A Closer Look at Argument Passing
- Returning Objects
- Arrays
- Understanding static keyword
- Introducing Nested and Inner Classes
- Using Command-Line Arguments
Inheritance
- Inheritance Basics
- Member Access and Inheritance
- A More Practical Example
- Using super
- Using super to Call Superclass Constructors
- A Second Use for super
- Creating a Multilevel Hierarchy
- When Constructors Are Called
- Method Overriding
- A Superclass Variable Can Reference a Subclass Object
- Dynamic Method Dispatch
- Why Overridden Methods?
- Applying Method Overriding
- Using Abstract Classes
- Using final with Inheritance
- Using final to Prevent Overriding
- Using final to Prevent Overriding
- Using final to Prevent Inheritance
- The Object Class
- toString() function
- equals() function
Packages and Interfaces
- Packages
- Defining a Package
- Path and CLASSPATH Settings
- A Short Package Example
- Access Protection
- Public, Private, Protected Access Specifies
- An Access Example
- Importing Packages
- Interfaces
- Defining an Interface
- Implementing Interfaces
- Difference and Similarities between Abstract classes and Interfaces
- When to use Abstract class and when to Interface
- Applying Interfaces
- Variables in Interfaces
- Interfaces Can Be Extended
- Runtime Polymorphism through Interfaces
Java Exception Handling
- Exception-Handling Fundamentals
- Exception Types
- Uncaught Exceptions
- Using try and catch
- Displaying a Description of an Exception
- Multiple catch Clauses
- Nested try Statements
- throw
- throws
- finally
- Java’s Built-in Exceptions
- Creating Your Own Exception Subclasses
- Chained Exceptions
- Using Exceptions
Multithreaded Programming in Java
- Multithreading basics
- Benefit of Multithreading
- The Thread Class
- Thread Priorities
- Implementing Runnable Interface to create Multiple threads
- Extending Thread class to create Multiple Threads
- Choosing an Approach
- Refined Runnable
- Using isAlive( ) and join( )
- Using Synchronized Methods
- The synchronized Statement
- Interthread Communication
- Deadlock
Input Output in Java
- I/O Basics
- Streams
- Byte Streams and Character Streams
- The Predefined Streams
- Reading Console Input
- Reading Characters
- Reading Strings
- Writing Console Output
- The PrintWriter Class
- Reading and Writing Files
The Collections Framework
- Collections Overview
- The Collection Interface
- The List Interface
- The Set Interface
- The SortedSet Interface
- The Collection Classes
- The ArrayList Class
- The LinkedList Class
- Accessing a Collection via an Iterator
- Using an Iterator
- Storing User-Defined Classes in Collections
- Working with Maps
- The Map Interfaces
- The Map Classes