Spring MVC Interview Questions and Answers
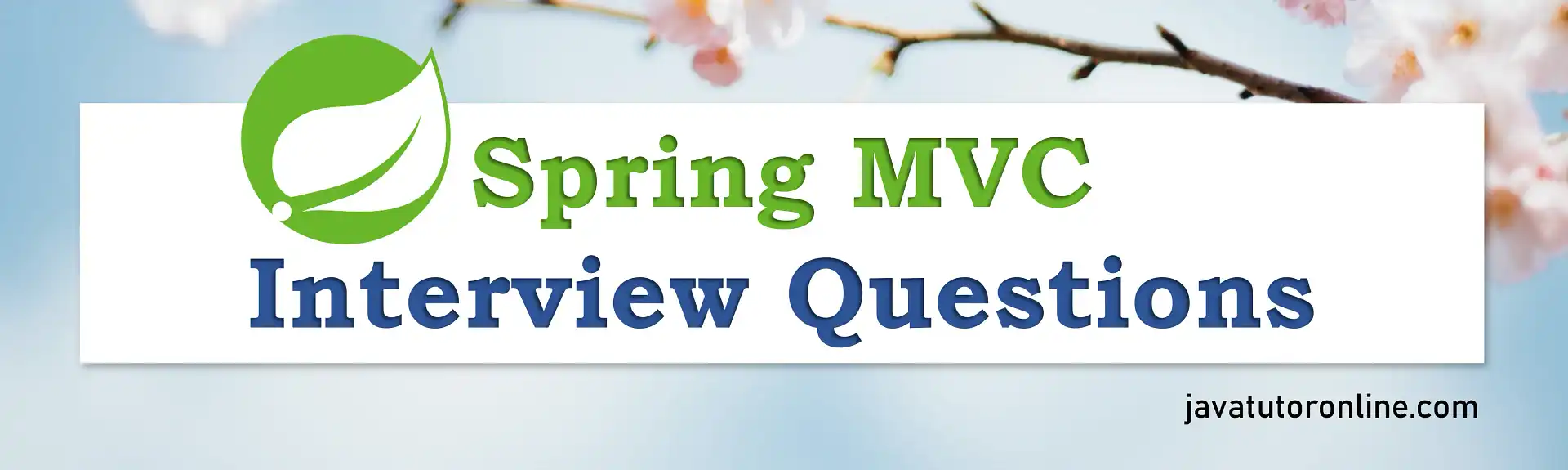
Preparing for a Java developer interview and looking for common Spring MVC questions? You’re in the right place! Spring MVC is a key part of the Spring Framework, making it easier to build web applications with features like the model-view-controller design, smooth request handling, and seamless integration with other Spring tools.
In this article, we’ll go through some of the most important Spring MVC interview questions to help you feel confident, whether you’re just starting out or already have experience with Spring MVC.
1. What is Spring MVC, and how does it work?
Spring MVC (Model-View-Controller) is a framework within the larger Spring Framework that helps in building Java-based web applications. It follows the MVC design pattern, which separates the application into three main components based on their roles.
- Model: It handles the application’s data and business logic, interacting with the database and preparing the data before showing it in the view.
- View: It manages the presentation layer by generating the user interface (e.g., HTML, JSP) to display data from the model to the user.
- Controller: It sits between the model and view components and is responsible for handling incoming requests, calling necessary services, and generating the right view to display as a response.
For a deeper understanding of Spring Mvc, consider enrolling in our Online Spring Mvc Training
2. Explain the Dispatcher Servlet role in Spring MVC.
In Spring MVC, the DispatcherServlet acts as the front controller. It is responsible for receiving requests and sending them to the appropriate handler method in the controller. After the controller processes the request and returns the response, the DispatcherServlet takes it and forwards it to a ViewResolver to render the view (e.g., JSP or Thymeleaf template). If the response is in JSON format, the DispatcherServlet uses tools like Jackson to serialize the data and send it back to the client.
3. How does Spring MVC handle requests?
When a request comes to the server, it is first intercepted by the DispatcherServlet. The DispatcherServlet is responsible for handling all the incoming HTTP requests. It then delegates the request to the HandlerMapping, which maps the request to the appropriate handler method in the controller based on the URL and HTTP method. After that, the controller method processes the request using the HandlerAdapter and interacts with the DAO (Data Access Object) layer to retrieve or update data with the help of the service layer. Finally, the controller returns the response to the DispatcherServlet for any further processing.
4. What are the key components of the Spring MVC architecture?
The key components of the Spring MVC architecture are:
- DispatcherServlet: This is the core of the Spring MVC framework that dispatches requests to the appropriate controller based on the URL mapping.
- Controller: Once the request reach the controller, the http method processes the request, and returns a ModelAndView object back to the DispatcherServlet.
- Model: The model stores the application data in the database and is responsible for handling the business logic.
- View: It is responsible for rendering the model data to the user. Generally, these are JSP, Thymeleaf, FreeMarker, or another template engine.
- HandlerMapping: Used by the DispatcherServlet to map the request to the appropriate controller.
- ViewResolver: Resolves the view name returned by the controller to an actual View.
- ModelAndView: Its an object holding both the model data and the view name, which is returned by the controller.
5. What is the role of @RequestMapping
annotation?
The @RequestMapping annotation in Spring MVC is used to map HTTP requests to controller methods. It is a generic annotation that can handle any type of HTTP request just by defining the URL pattern and the request type (e.g., GET, POST) that the method should handle. This annotation can be applied to both classes and methods to handle various types of requests.
6. What are HTTP-specific annotations used for request handling?
Here’s the list of HTTP-specific annotations for handling requests in Spring MVC:
@GetMapping
- Used for handling GET requests, usually for those operations that retrieve resources from the server, such as fetching data or displaying a page.
@PostMapping
- Used for handling POST requests, typically for the operations that create a new resource on the server or submit data for processing.
@PutMapping
- Used for handling PUT requests, generally for operations that replace or update a resource on the server, such as updating all the data of an existing resource.
@PatchMapping
- Used for handling PATCH requests, usually for operations that partially update a resource on the server, such as updating specific fields of an existing resource.
@DeleteMapping
- Used for handling DELETE requests, generally for operations that remove a resource from the server, such as deleting a record or resource.
7. How do you handle form submissions in Spring MVC and Spring REST?
Spring MVC
When a user submits a form with an HTTP POST request, it is processed by the handler method in the controller, usually using @PostMapping
. Then the form data is automatically bound to a DTO (Data Transfer Object) using @ModelAttribute
. After the data is bound, it is validated using @Valid
. Once the data is bound and validated, the controller processes it and then performs necessary actions like saving it to the database.
Spring REST
For RESTful APIs, when a user sends a POST request, the data is typically passed in the request body, usually in JSON format. The form data (or JSON body) is automatically bound to a DTO (Data Transfer Object) using @RequestBody
. After binding, the data is validated using @Valid
. The controller then processes the data, performing any necessary operations like saving to the database.
8. What is the role of HandlingMapping and HandlerAdapter interface in Spring?:
The HandlerMapping interface is responsible for mapping an HTTP request to the appropriate handler (controller method). It determines which controller method should handle the request based on the URL pattern and HTTP method type
So, when a HTTP request arrives –
- The DispatcherServlet intercepts the request and passes it to the HandlerMapping to determine which controller method should handle it.
- The HandlerMapping checks all configured controller methods and finds the right one based on the request’s URL pattern and HTTP method.
- Once determined, HandlerMapping returns the mapped handler in form of HandlerExecutionChain back to DispatcherServlet.
- Now, the DispatcherServlet use this mapped handler to invoke the controller method that matches the request with the help of HandlerAdapter.
- The HandlerAdapter also passes any required arguments from the HTTP request (like query parameters, path variables, etc.).
9. How can we configure the DispatcherServlet in a Spring MVC application?
There are two common ways by which we can configure out Spring MVC web application.
- Configure the
DispatcherServlet
manually inweb.xml
XML file, defining the servlet and its URL pattern. This is the traditional way to configure the DispatcherServlet. - Another way is using Spring Boot, where the
DispatcherServlet
is auto-configured without the need forweb.xml
. This is the modern way to do and it use Java-based configuration.
10. What is the role of @Controller
and how can we create one in Spring MVC?
We use the @Controller annotation in Spring MVC to define a class that handles HTTP requests and returns appropriate responses. It interacts with the Model using the Service layer to process data and return a View along with its model data. The @Controller annotation is a stereotype annotation, meaning that Spring will automatically detect and manage this class as a bean during component scanning.
This annotation is used in request handler methods marked with @GetMapping, @PostMapping, etc like annotations.
We hope this list of interview questions and answers has helped you understand Spring MVC better. Make sure to keep learning, try out real examples, and stay updated with the latest changes in Spring MVC. All the best for your interview, and happy coding!
Acknowledgments
The questions and answers in this article were inspired by the official Spring documentation, particularly from Spring MVC Reference. A big thank you to the Spring team for their amazing resources that helped in putting this list together.
Leave a Reply